Onboarding - The Volcano Plot
Welcome! This guide will teach you how to get started with Python and The Materials Project API. The Materials Project is a powerful database providing access to over 140,000 unique materials. These materials along with a plethora of their properties were calculated ab-initio, or from first principles. Many of these calculations are driven by high-throughput density-functional theory (DFT). Given only a materials crystal structure, DFT can calculate a variety of different materials properties. By the end of this guide you’ll have learned to (1) run Python scripts, (2) connect to the Materials Project API, and (3) plot in matplotlib. This will be done by creating what we like to call “The Volcano Plot” from the paper, “A map of the inorganic ternary metal nitrides”, by Wenhao Sun et al.
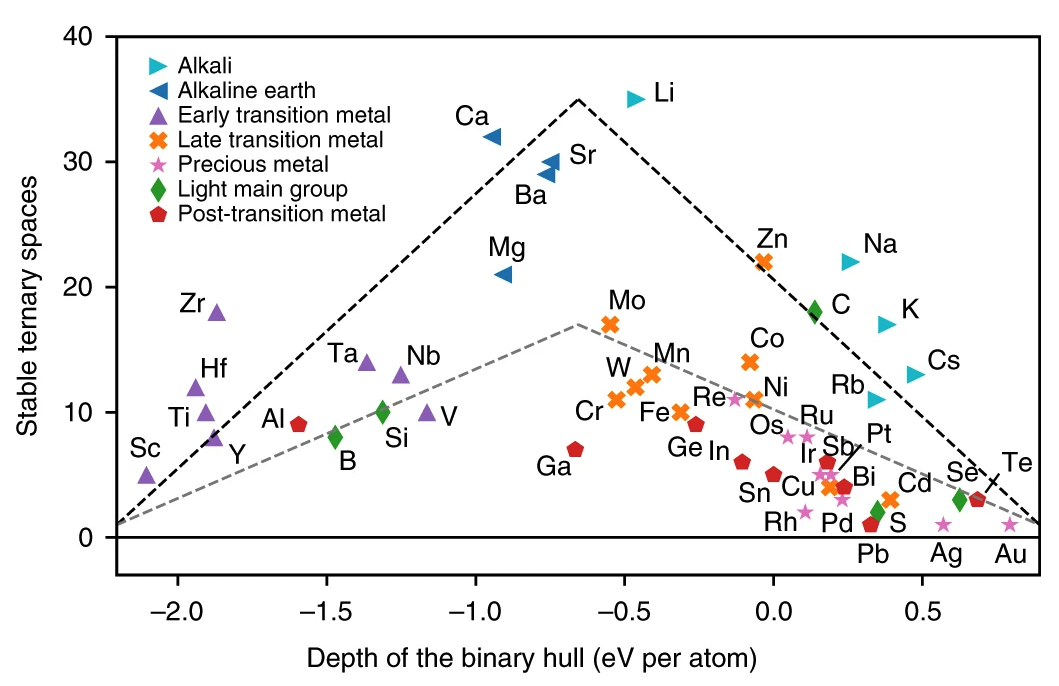
Getting started with Python
There are many different guides online for getting started with Python. This guide will use Anaconda for managing Python environments and Visual Studio Code as an intergrated development environment (IDE).
1. Setting up a conda environment
Download and install Anaconda. Follow the installation instructions for your OS. Open a new terminal and create a new environment using the conda create --name myenv
command. Replace myenv
with your desired environment name. Now to navigate to your new environment use conda activate myenv
and to naviagate away use conda deactivate myenv
. Now your ready to use Python! Simply navigate to your new environment and type python
to enter the interactive interpreter.
2. Setting up Visual Studo Code (VSCode)
Download and install Visual Studo Code. Follow the installation instructions for your OS. VSCode is a lightweight IDE used for a variety of langauges. Once installed open VSCode and navigate to the “Extensions” tab. Search the marketplace for the “Python” extension and install. Once installed VSCode will be able to recognize Python, giving you meaningful feedback from the IDE when writing your code.
Now locate the “New File” option in VSCode to create a new file. Then save it as a .py
file to tell VSCode that this file is a python script. Now in VSCode open a “New Terminal” using the toolbar. From here you should have opened a new interactive shell within VSCode. Now active your conda environment and type python filename.py
to run your script (make sure your in the same directory as your saved python file).
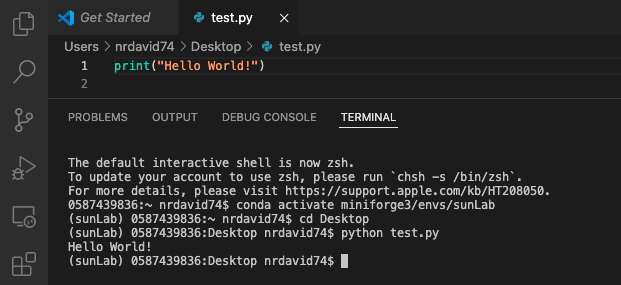
At this point it’s also a good idea to open the Command Palette to tell VSCode (and Pylance) which Python interpreter to point to. This will allow VSCode to properly identify which packages you have installed and which you do not. Use ⇧⌘P (Mac) or Ctrl+Shift+P
(Windows). Then type Python: Select Interpreter
and select the option. From here you should see all your available global environments. Choose the one you’ve setup and are using.
Connecting to the Materials Project API
The Materials Project allows anyone to have direct access to current, most up-to-date information for the Materials Project database through their application programming interface (API).
1. Getting a Key
Go to the Materials Project API and create an account to acquire a key. This key is your API access to their database. Keep track of this.
2. Connecting to the API
Since you’ve created a new conda environment, you’ll need to install the required packages in order to connect to the Materials Project API. This can be done via conda install <package name>
. In your environment type:
conda install mp-api
into your terminal. After doing open a new python file and run the following code:
# Testing connectivity to API by grabbing data for "mp-149"
from mp_api import MPRester
with MPRester(api_key=YOUR_KEY) as mpr:
doc = mpr.summary.get_data_by_id("mp-149")
If the code above executes with no errors, you’re all set!
Completing the Onboarding Assignment
Now you have all the necessary ground work to produce the volcano plot! The next steps are entirely up to you, but I would recommend following the ordered list below.
- Read “A map of the inorganic ternary metal nitrides”, by Wenhao Sun et al.
- Read the Materials Project API Docs and play around with the API functionality.
- Acquire the data needed to create the volcano plot.
- Familiarize yourself with matplotlib, then make the plot! Note: The plot may look slightly different from API changes over the years.
Lastly, good luck!